JavaScript, HTML, and CSS
JavaScript and its relationship to HTML and CSS
JavaScript is a powerful language that is lightweight and can communicate with the browser.JavaScript programs should be stored in and delivered as .js files. Javascript is different from "Java".JavaScript, HTML, and CSS are the foundational technologies for building websites.JavaScript is like the soul of the human body.HTML and CSS are the body and the appearance. JS brings the body to life!. Using the analogy of switchboard and wiring, we could understand how they work together.
* HTML is the The Switchboard. It is the physical structure where switches, buttons, and labels are placed. It represents the content and structure of the webpage.The buttons and bulb are like the physical components on a switchboard.
* CSS is the design of the Switchboard.It determines its appearance. how the wires and switches look and makes it visually appealing.Basically a user-friendly Switchboard design.
* JavaScript is doing the wiring functionality. It enables the switches to perform actions like turning the light bulb on or off. These wires connect buttons to the bulb, without it the switchboard would just be a static display, making it functional.
Similarly,Remote and TV do too. The TV represents the structure like HTML does. Design of the TV determines its style, that is our CSS. Javascript is the Remote Control, which allows you to interact with the TV.It connects the remote to the TV , enabling functionality like turning it on, off, and adjusting the volume.
We can find elements by class name and by ID using . and #, respectively (just like with CSS), or by combining these methods, for example: If we wanted to find the first element with the 'w3schools-tutorial' id we would use: document.querySelector('#w3schools-tutorial') If we wanted to find the first element with the 'w3schools-tutorial' id we would use: document.querySelector('a#w3schools-tutorial') See the difference? We've got an 'a' in front of the '#' to indicate that we only care about elements. If we wanted a list of all elements with the 'dom' class we would use: document.querySelectorAll('.dom')
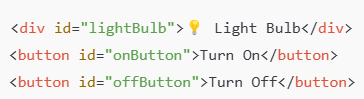
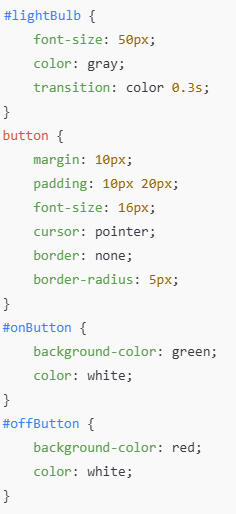
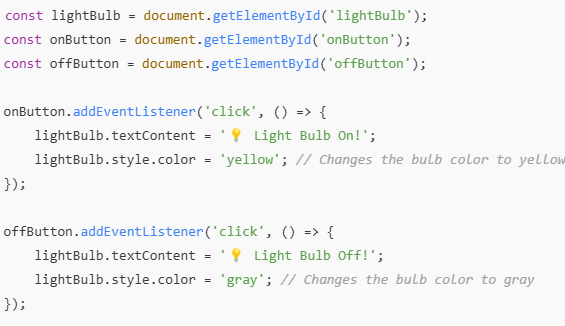
Control flow and loops using an example process from everyday life
Using the Example of doing laundry
Control Flow:
- If the laundry basket is full, start the laundry. If not wait until its full.
- If the washing machine is empty and clean,put in the clothes. If not, wait until the machine is ready.
- If the machine is ready, add appropriate detergents and softeners and then start the machine.
- If the clothes are clean, move them to the dryer, if not wait until it finishes.
- If the dryer is done, fold the clothes. If not, wait for it to finish.
Loop:
-
Checking the laundry load
- Start washing a load.
- Repeat the process,If clothes are not clean
- Repeat the step for each load of laundry, Until all clothes are washed and dried.
DOM and an example of how you might interact with it
The Document Object Model (DOM), generally abbreviated to DOM, is the way through which JavaScript interacts with content within a website.The DOM is not a programming language, but without it, the JavaScript language wouldn't have any model or notion of web pages, HTML documents, SVG documents, and their component parts.The DOM is not part of the JavaScript language, but is instead a Web API used to build websites. For example, Node.js runs JavaScript programs on a computer, but provides a different set of APIs, and the DOM API is not a core part of the Node.js runtime.
What your browser displays is a web document.what you see is a collision of HTML, CSS, and JavaScript working together to create what gets shown.Digging one step deeper, under the covers, there is a hierarchical structure that your browser uses to make sense of everything going on.Browsers provide a document JavaScript object with a large number of properties and methods to allow us to do precisely this. It's a "tree" of objects, with JS objects representing the head, body, title, paragraphs, etc. It's like a family tree, except each object, method, or property has only one parent. At the head of this tree-the great-great-great-grandparent of all the rest, so to speak - the original ancestor - is the window object, and inside the window object is the document object, which represents our page.
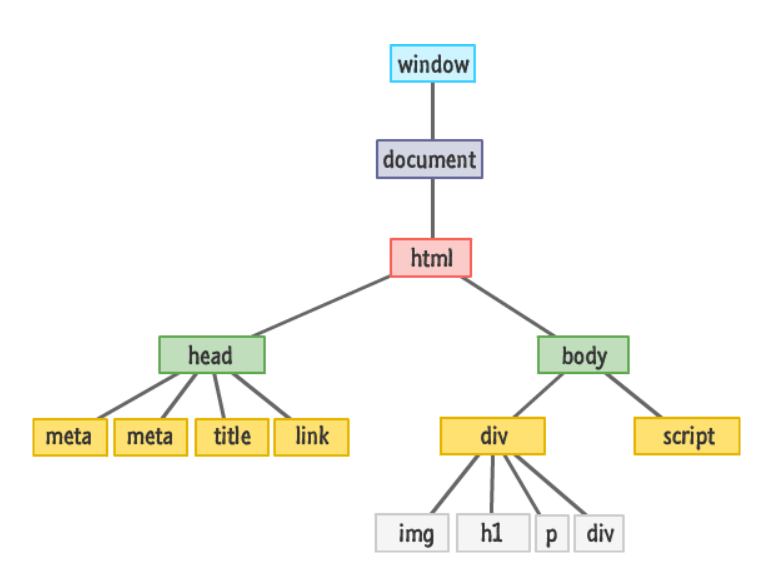
Everything that makes up your DOM is more generically known as nodes.These nodes can be elements (which shouldn't surprise you), attributes, text content, comments, document-related stuff, and various other things you simply never think about. That detail is important to someone, but that "someone" shouldn't be you and me. Almost always, the only kind of node we will care about is the element kind because that is what we will be dealing with 99% of the time. The document object does not simply represent a read-only version of the HTML document. It is a two-way street where you can read as well as manipulate your document at will
Example of Interacting with the DOM
Imagine you have a web page with a button that changes the background color of a specific when clicked.
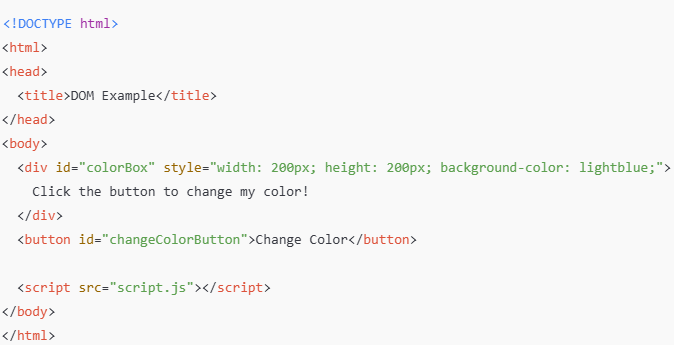
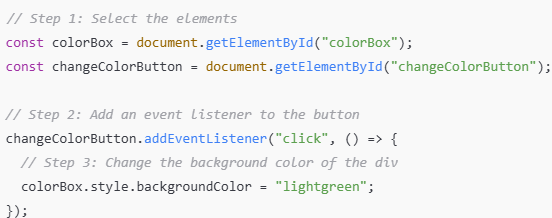
difference between accessing data from arrays and objects
An array is a special variable, which can hold more than one value. An array can hold many values under a single name, and you can access the values by referring to an index number.Using an array literal is the easiest way to create a JavaScript Array.The length property of an array returns the length of an array. Syntax: const array_name = [item1, item2, ...];
const cars = ["Saab", "Volvo", "BMW"];
Array indexes start with 0. [0] is the first element. [1] is the second element.The JavaScript method toString() converts an array to a string of (comma separated) array values.
const fruits = ["Banana", "Orange", "Apple", "Mango"]; document.getElementById("demo").innerHTML = fruits.toString();
With JavaScript, the full array can be accessed by referring to the array name.
const cars = ["Saab", "Volvo", "BMW"]; document.getElementById("demo").innerHTML = cars;
The length property is always one more than the highest array index.
- Arrays are ordered collections of data (indexed numerically).
- const fruits = ["apple", "banana", "cherry"];
- console.log(fruits[0]); // Output: apple
- console.log(fruits[1]); // Output: banana
- Arrays are best when you need to store lists of similar data or when the order of items matters.
Objects: data type containing unordered (but labeled) key/value pairs, stored within curly brackets {}. Each 'key' acts as a label for the corresponding 'value'. Key/value pairs are referred to together as a 'property'.
const popcorn = { foodType: 'movie snack', price: 4.99, flavour: 'salt' }
Above, popcorn is the name of the object. foodType is a 'key', 'movie snack' is a 'value', and together this key/value pair is referred to as a 'property'. There are multiple ways to access or edit data from inside of an object. One common way to retrieve data from an object is by using dot notation, where we say the name of the object, followed by a dot and then the key we would like to access. For example if we wanted to know the price of popcorn we could use popcorn.price, which provides the value of 4.99. While popcorn.flavour would provide the value of 'salt'.- Objects are unordered collections of key-value pairs.
- Dot notation: object.key
- Bracket notation: object["key"]
- const car = { brand: "Toyota", model: "Corolla", year: 2020 };
- console.log(car.brand); // Output: Toyota (dot notation)
- console.log(car["model"]); // Output: Corolla (bracket notation)
- Objects are better for storing related data with descriptive keys (e.g., properties of an entity).
What functions are and why they are helpful
A function is a block of reusable code designed to perform a specific task. Functions take inputs (optional), process them, and return an output (optional).After declaring/writing a function, you'll want to use that function! Do that by 'calling' it. You call a function by writing its name, followed by brackets (). functions do not run without calling them.
Function keyword, followed by an identifier/name of the function, then brackets which optionally contain parameters separated by commas, then curly brackets inside of which is the statement(s) that define the function. A piece of code typically used to execute a task, written so that it can be called upon multiple times if needed.
Features of functions are:-
- Reusability: It allow us to reuse the same code multiple times. It helps to avoid code duplication. That means, It overcome the repetition problem.
- Avoid Repetition (DRY Principle)
- Parameters and Return Values : you can pass inputs into functions and receive outputs, It makes them flexible and powerful.
- Maintability: It's easy to debug and understand.